Cango3D User Guide
Cango3D canvas graphics library
This is the user guide for the Cango3D canvas graphics library. The current version of Cango3D is 14v03, and the source code may be downloaded at Cango3D-14v03.js and the minified version available at Cango3D-14v03-min.js. For an introduction to Cango3D see Cango3D Graphics Library page.
New in Version 14
- Dynamic transform methods are now only available on Group3D. Transforms for the Path3D are now only the 'hard' transforms set from parameters passed to the constructor or by the setProperty method. They are not reset after rendering as are Group3D transform methods.
- Drag and Drop behaviour has been refactored to simplify writing event handlers. The eventData object has been given revised properties to better support roll, pitch and yaw transforms. This refactor has also removed the need for the 'grabTransformRestore' method further simplifying event handlers.
New in Version 13
- Three new rotation transform methods yaw, pitch and roll have been added to Group objects. These transforms rotate the object with reference to the object's own, intrinsic coordinate axes that rotate with the object. The other rotation transform methods 'rotateX', 'rotateY' and 'rotateZ' rotate an object with reference to the fixed world coordinate axes.
New in Version 12
- Extension Object support has been added. Extension objects are a sub-class of the Group3D but are distinguished by supporting preSort and preRender methods which can access the properties and methods of the Cango3D instance doing the rendering.
- The Cango3D object has the wireframe method added. It behaves the same as 'render' but the Shape3D objects have only their outline paths drawn, as a consequence the render method 'wireframe' parameter has been dropped.
Cango3D architecture
Cango3D is based on the fact that straight lines and Bézier curves maintain their shape when 3D transform such as rotation, scaling and translation are applied and the fact that the HTML canvas element has native methods for drawing both straight lines and Bézier curves. So Cango3D bases all its drawing on one class, the Path3D.
Path3D
The Path3D class is the basis of all drawing done by Cango3D. Path3D objects can be rendered by any Cango3D instance. Path3D properties hold the definition of an outline path defined using SVG path syntax for 'move', 'line', 'cubic Bézier' and 'close path' commands with 3D coordinates. It also has properties holding various style values that determine the appearance of the path when rendered.
Path3D have transform properties that alter the orientation, size and offset from the origin of the path. This allows the path to be rendered and re-rendered to the canvas without having to be reconstructed every time.
Shape3D
Shape3D objects are a sub-class of Path3D and are also considered as primitives that are rendered to the canvas. A Shape3D object path definition is always a closed and always filled with color when rendered. Shape3D support additional properties such as fillColor, backColor, backHidden etc. The fillColor can be flat or gradient, flat colors are shaded according to the location of the light source defined within the Cango3D instance that is rendering the object.
Group3D
To draw arbitrarily complex 3D objects, Cango3D provides the Group3D class which holds an array of child objects, either Path3D or more Group3D forming a family tree of Path3D (or Shape3D) leaf elements to be rendered to the canvas. The descendant Path3D may manoeuvered as a single entity using the Group3D transform methods.
Group3D have dynamic transform methods such as translate, rotate and scale etc. Any of these transforms that have been applied to Group3Ds are inherited by the groups' children recursively and finally the net accumulated transforms are applied to the leaf Path3D. The transforms are effected by applying the corresponding transform matrix to the Path3D outline coordinates. See Cango3D Transforms for details and examples.
Cango3D
The Cango3D object itself has methods to establish a world coordinate system that will map the Path3D coordinates to canvas pixel values. Its 'render' method does all the coordinate transforms, projection to 2D and mapping to pixels and final conversion to canvas 2D drawing methods.
Extension Objects
Cango3D supports Extension objects, which have additional optional methods, 'preSort' and 'preRender' which run with knowledge of the Cango3D instance that is rendering them. The optional 'preSort' method enables modification to group and object properties and transforms prior to sorting into the order of rendering and hence which objects will overlay others. The optional 'preRender' method enables modification to Path3D and Shape3D object properties and transforms after sorting, immediately prior to rendering. Several Extension Objects are included in the Cango3D core library. These are:
- Text3D a string of characters in the Hershey stroke font rendered as a group of Path3D objects.
- Panel3D objects have a child Shape3D, initially defined by 2D SVG syntax data in the XY plane. Panel3D may have Text3D objects applied as labels which always rendered with the front face of the Panel3D.
- Sphere3D is a pseudo 3D object, actually a circular Shape3D rendered with shading and a specular refection highlight dependent on the position of the object relative to the light source and observer.
- ObjectByRevolution3D is an object defined by a series of SVG (2D) commands defining a path in the XY plane. Copies of the path are rotated about the Y axis in a user defined number of steps. Adjacent pairs of these paths are then joined with ars or straight lines forming a Shape3D. The group of Shape3D form the ObjByRevolution3D.
- ObjectByExtrusion3D a closed object with a Panel3D at each end. Each segment of the end panel outline path is joined to the corresponding segment of the other end by straight lines defining a Shape3D face. The group of faces and ends form the ObjectByExtrusion3D.
- SurfaceByExtrusion3D is an open object with its front edge defined by a Path3D, the back edge is a copy of this path. Each segment of the Path3D is joined to the corresponding segment of the back Path3D by straight lines defining a Shape3D face. The group of faces form the SurfaceByExtrusion3D.
Rendering
The principal method used to draw Path3D onto the canvas is Cango3D.render. The render method takes either a Group3D or a Path3D as its first parameter. In the case of Group3D, all transforms that have been applied to it are inherited by its children. Child Group3D add all inherited transforms to any transforms applied to it directly and the net transform is then propagated recursively its descendants and finally to the leaf Path3D. The Path3D objects, with transforms applied, are then depth sorted and projected into 2D. The canvas is cleared and all the Path3D rendered to the canvas.
The Cango3D.wireframe method behaves just as 'render' but without filling any Shape3D objects with color.
Both 'render' and 'wireframe' can accept a second optional parameter, if this is the string "noclear", the canvas is not cleared prior to rendering, all other values are ignored.
The Path3D object has a paint method that then makes the low level calls to the canvas native drawing methods. For more complex applications this 'paint' method can be replaced by a user defined function. An example of this can be seen in use on the Rotating Globe page.
Cango3D
Syntax:
const cgo = new Cango3D(canvasID);
Description:
To start drawing an instance of the Cango3D graphics context must be created. This requires a canvas element to exist in the web page and for it to have a unique ID. Pass the canvas ID to the Cango3D constructor and the Cango3D object is returned.
Parameters:
canvasID: String - The 'id' attribute of the canvas object on which the Cango3D graphics context will draw.
Returns:
Cango3D object - An instance of the Cango3D graphics library which has all the following methods and properties.
Cango3D methods
Syntax:
cgo.setWorldCoords3D(lowerLeftX, lowerLeftY, spanX);
Description:
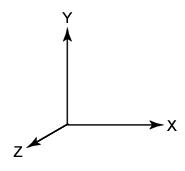
Defines a world coordinate system for subsequent drawing operations with this graphics context. The world coordinate system sets translation and scaling factors to map world coordinate values to canvas pixel coordinates.
The coordinate system is Right Handed Cartesian, with X values increasing to the RIGHT, Y increasing UP the screen and Z increasing OUT from the screen. The canvas always lies in the XY plane at Z=0. The viewpoint is at some distance from the canvas along the positive Z axis. The distance determines the field of view (FOV).
World coordinates are set by defining the coordinate values of the lower-left corner and width of the canvas. Since the canvas has equal scaling factors in the X, Y and Z directions, setting the span of the canvas X dimension is sufficient to set all scaling factors. The X,Y coordinates of the lower-left corner are sufficient to set the canvas pixel location of the world coordinate origin (0,0,0). The lower-left Z coordinate value does not need to be specified, it is always 0 as the canvas is always in the XY plane.
Parameters:
lowerLeftX: Number - World X coordinate value of the lower left corner of the canvas.
lowerLeftY: Number - World Y coordinate value of the lower left corner of the canvas.
spanX: Number - The width of the canvas in world coordinates.
If setWorldCoords3D is called with no parameters passed, or not called at all, the world coordinate system is set to have the canvas lowerLeft=(0,0) and spanX = 100.
Syntax:
cgo.setLightSource(x, y, z);
Description:
Defines a vector in 3D space starting at the origin and ending at (x, y, z). The shading of the fill color of Shape3D objects is determined by the dot product of the unit vector in the direction of the light source and the Path3D normal (the unit vector normal to the front face of the object). If no call is made to setLightSource the default value is (x=0, y=100, z=500).
Parameters:
x, y, z: Numbers - the X, Y, Z coordinates of a vector from the origin in the light source measured in world coordinates.
Syntax:
cgo.setFOV(deg);
Description:
This method sets the Field Of View. The is effected by calculating the location of the viewpoint at which the width of the canvas will subtend an angle of 'deg'. The viewpoint distance is used for all subsequent rendering on the canvas. The Field Of View will determine the extent of perspective distortion of an objects dimensions. The setFOV method restricts the value of 'deg' to less than 60°, since values greater than 60° produce less realistic perspective effects. A value of 45° is recommended. A value of 0° would place the viewpoint at infinity removing perspective effects. The calculation assumes the world coordinate origin is within the field of view ie. on the canvas, if not, the field of view is calculated as if the origin were on the edge of the canvas.
Parameters:
deg: Number - The angle subtended at the viewpoint by the width of the canvas, measured in degrees. The viewpoint distance along the Z axis is set to yield the requested Field Of View. Angles greater than 60° are ignored.
Syntax:
cgo.setPropertyDefault(propertyName, val);
Description:
Sets the default values for various system properties. The property whose default is to be set is specified by the string 'propertyName' this string is not case sensitive and may take the values set out in column 1 of the table below.
The new default value is passed as 'val'. Allowed values are restricted to the range appropriate to the property. If the property is not listed or the value is an incorrect type or outside the allowed range, then the call is ignored and the current default value remains unaltered.
System defaults for all the properties are listed in column 4.
Name | Type | Range | Default |
---|---|---|---|
backgroundColor | CSS Color | - | set by canvas element style |
fillColor | CSS Color | - | 'rgba(128, 128, 128, 1.0)' |
backColor | CSS Color | - | 'rgba(128, 128, 128, 1.0)' |
strokeColor | CSS Color | - | 'rgba(0, 0, 0, 1.0)' |
strokeWidth | Number (pixels) | - | 1 |
lineCap | String | 'butt', 'round', 'square' | 'butt' |
lineJoin | String | 'bevel', 'round', 'miter' | 'round' |
Parameters:
propertyName: String - The name of the property whose default value is to be set. It must be one of the strings listed in column 1 of the table above. The string value is not case sensitive.
value: various - The new default value for the property. Its type should match the type listed in column 2 of the table above and the range of values must fall within the range listed in column 3.
Syntax:
cgo.render(obj [, "noclear"]);
Description:
This method renders a Path3D or all the Path3D descendants of the Group3D, onto the canvas. By default the render method first clears the canvas. The optional string parameter 'noclear' is supported. This which will inhibit the canvas being cleared so the object will be rendered in addition to the drawings already on the canvas.
The 'render' method recursively propagates the root group transforms down through the descendent Groups to all the leaf Path3D objects. All Path3D are then sorted into the order that they will be drawn, based on distance of the Path3D's centroid from the viewpoint, most distant (smallest Z coordinate value) will be drawn first. The all the descendant Path3D within each Group3D are sorted among themselves. All coordinates of the Path3D are then projected from 3D to 2D to create the native 2D canvas draw commands which are then applied to the canvas.
Parameters:
obj: Group3D (or single Path3D) - The group or object to be rendered to the canvas.
"noclear": String (optional) - If the string 'noclear' is passed as a parameter the canvas is not cleared prior to rendering.
Syntax:
cgo.wireframe(obj [, "noclear"]);
Description:
This method renders a Path3D or all the Path3D descendants of the Group3D, onto the canvas. The behaviour is identical to the 'render' method except that 'wireframe' inhibits the color filling of all Shape3D.
Parameters:
obj: Path3D or Group3D - The object or group of objects to be rendered to the canvas.
"noclear": String (optional) - If the string 'noclear' is passed as a parameter the canvas is not cleared prior to wireframe drawing.
Syntax:
cgo.clearCanvas();
Description:
Clears the canvas, deleting all drawing. The canvas filled with the Cango3D context default background-color which can be set by the 'setPropertyDefault' method as follows:
cgo.setPropertyDefault("backgroundColor", "color string");
The color string may take any of the valid HTML color formats eg. "pink", "rgb(128, 120, 40)", etc. see Colors.
If the default color has not been explicitly set the canvas will be filled with the canvas element 'background-color'. The background color may be set by CSS style properties of the canvas element. Listening for mousedown events on any draggable objects is canceled.
Parameters:
none
Syntax:
cgo.initZoomTurn(obj);
Description:
Creates an transparent overlay canvas and draws a set of button-like controls in the top right hand corner. The buttons are enabled to respond the mouse click event. The + and - will reset the world coordinates so that the span of the canvas is reduced or increased by 20% per click. The object is then redraw the object giving the effect of zooming in or out. The <, >, ^, v will redraw the object rotated by 5° to the left, right, up or down respectively. The x button redraws the object at the original zoom and turn values.
Note: initZoomTurn requires the CanvasStack-2v01.js module to be loaded.
Parameters:
obj: Path3D or Group3D - The object or group that will be re-drawn for each zoom or turn step.
Example:
The figure below shows an ObjectByRevolution3D forming a drawing of a wine glass. The Congo3D initZoomTurn has been used to allow a user to zoom and turn the drawing to see the object from any desired aspect. The source code for this simple example is as follows:
function zoomDemo(cvsID) { const glass = "M 118,0 c 0,5.71 -0.25,11.05 -1.89,15.69 -1.64,4.63 -4.69,8.53 -10.24,11.44 \ -21.68,11.40 -52.93,20.24 -68.17,26.25 -7.61,3 -14,6.24 -19,11.87 \ -5,5.62 -8.61,13.63 -10.66,26.16 -1.63,10 -3.85,75.22 1.42,82.85 \ 3.45,4.98 5.68,8 29.64,13.85 10,2.41 19.63,3.28 22.49,6.85"; const g3 = new Cango3D(cvsID); g3.setWorldCoords3D(-250, -250, 500); g3.setLightSource(-200, 200, 300); const glassObj = new ObjectByRevolution3D(glass, 24, false, { fillColor:"yellow", backColor:"lightGreen"}); g3.initZoomTurn(glassObj); }
Cango3D public properties
Property | Type | Description |
---|---|---|
ctx | CanvasRenderingContext2D | The raw canvas drawing context. This is available to access the more esoteric canvas capabilities. |
aRatio | Number | Aspect ratio of the canvas element, the canvas width divided by canvas height, eg. if the canvas is 300px wide and 200px high, cgo.aRatio = 1.5. |
xscl | Number | Scale factor converting World Coordinates to canvas pixels. All three axes X,Y and Z have this scaling applied prior to the 3D to 2D projection. |
Path3D
Path3D objects and and the sub-class Shape3D may be rendered and transformed and re-rendered by any Cango3D instance without having to be re-constructed.
Syntax:
const pth = new Path3D(descriptor, options);
Parameters:
descriptor: Array - An array of Cgo3D format commands and their associated 3D coordinates defining the path.
When rendered, a Path3D outline will be drawn with the line width set by the 'lineWidth' (in pixels) or 'lineWidthWC' (in world coordinates) property. The line color is set by its 'strokeColor' property. If these properties has not been set explicitly by on the 'options' object or by its 'setProperty' method, then the default value used is taken from the Cango3D context used to render the Path3D.
options: Object - The key-values pairs define the values of the various properties of the Path3D the supported keys are listed in the Path3D and Shape3D properties section.
Syntax:
const shp = new Shape3D(descriptor, options);
Parameters:
descriptor: Array - An array of Cgo3D format commands and their associated 3D coordinates defining the outline of the shape.
When rendered a Shape3D outline path will be closed (if not already closed by the "Z" command) and the filled with color. If the normal to the surface is facing toward the viewpoint, it is filled with color value of its 'fillColor' property, if facing away it is filled with 'backColor'. If either of these properties have not been set explicitly by the user code then the default values from the Cango3D context rendering the object will be used. If it is facing away from the observer and the property 'backHidden' is set 'true' then the shape will not be rendered. This behaviour is used for closed 3D objects where the interior walls are never seen so only the outward facing shapes need be rendered.
options: Object - The key-values pairs define the values of the various properties of the Shape3D the supported keys are listed in the Path3D and Shape3D properties section.
Path3D and Shape3D descriptors
The Path3D and Shape3D constructors take as their descriptor an array of commands defining their outline. The command syntax is referred to as Cgo3D format. Outline data is often imported in 2D SVG format, perhaps copied from an SVG editor such as InkScape, or as a PathSVG module which provides the outline path of many commonly used shapes and utility methods to transform them. These 2D data must first be converted to Cgo3D before being passed to the Shape3D constructor. The svgToCgo3D utility is provided to do this conversion.
svgToCgo3D converts the full 2D SVG command set into the Cgo3D set of commands and translates any relative commands and coordinates into absolute and a Right Handed Cartesian (RHC) coordinate system. To do this it translates the 'A' (draw arc) and other non-Cgo3D commands to Bézier curves and straight lines. It also SVG Left Handed coordinates by flipping the sign of all Y coordinates and the sign of the angles if the 2D data is passed as a String object. If the data is passed as an Array object then the coordinates are assumed to be calculated in RHC coordinates which have the Y coordinates +ve up the screen and angles positive counter-clockwise and so don't need to be changed.
The Cgo3D format consists of a series of single letter draw commands and their associated x,y,z coordinate values. The commands are single letter strings; "M", "L", "C", "Z". A subset of the SVG path commands. Cgo3D commands are case-sensitive, uppercase commands take absolute coordinates measured from the world coordinate origin (0,0,0). Lowercase commands coordinates are measured relative to the current pen position ie. the end point of previous command. The first point must always be a 'M' (move) command using absolute positioning as there is no previous pen position.
As an example of Cgo3D format, here is the array of commands to draw a unit square in the XY plane with the drawing origin (0,0,0) at it's center.
const square = ['M', 0.5,-0.5,0, 'l', 0,1,0, -1,0,0, 0,-1,0, 'z'];
The syntax is a sub-set of the SVG path drawing command set, restricted to straight lines, Quadratic and Cubic Bézier curve drawing commands.
Cgo3D command set
Command |
Parameters |
Description |
---|---|---|
M |
x, y, z |
moveTo: Moves the pen to a new location. No line is drawn. All path data must begin with a 'moveto' command. |
Line Commands |
||
L |
x, y, z |
lineTo: Draws a line from the current point to the point (x,y,z). |
Cubic Bézier Curve Commands |
||
C |
x1, y1, z1, x2, y2, z2, x, y, z |
bezierCurveTo: Draw a cubic Bézier curve to the point (x,y,z) where the points (x1,y1,z1) and (x2,y2,z2) are the start and end control points, respectively. |
End Path Commands |
||
Z |
- |
closePath: Closes the path. A line is drawn from the last point to the first. |
Path3D and Shape3D properties
The following tables set out all the supported object properties that may be set as key-value pairs passed to the constructor or set individually after construction using the 'setProperty' method.
Path3D Style properties
Property Name | Type | Range / Description | Path3D | Shape3D (applied to border) |
---|---|---|---|---|
strokeColor | CSS Color | Color used to render a Path3D or the border line of a Shape3D if border=true. | ✓ | ✓ |
lineWidthWC | Number (World Coords) | Width of the Path3D outline or Shape3D border when rendered, measured in world coordinates. | ✓ | ✓ |
lineWidth | Number (pixels) | Width of the Path3D outline or Shape3D border when rendered, measured in pixels. | ✓ | ✓ |
lineCap | String | 'butt', 'round', 'square' | ✓ | ✓ |
Shape3D additional style properties
Property Name | Type | Range / Description | Path3D | Shape3D |
---|---|---|---|---|
fillColor | CSS Color | Color saturation is set by the angle to the light source. | ✓ | |
backColor | CSS Color | Color seen when the backHidden=false and the normal is pointing away from the viewpoint (normal.z < 0). | ✓ | |
flatColor | Boolean | If 'true' the 'fillColor' saturation is independent of the angle to the position of the light source. | ✓ | |
backHidden | Boolean | If true the Shape3D is not filled with color if the normal is pointing away from the viewpoint. Used for drawing closed volumes. | ✓ | |
border | Boolean | true or falsy | ✓ |
Transform common properties
Property Name | Type | Range / Description |
---|---|---|
x | Number (World Coords) |
The offset along the X axis by which the object is moved relative to its drawing origin (0, 0). This offset is permanent and cumulative. |
y | Number (World Coords) |
The offset along the Y axis by which the object is moved relative to its drawing origin (0, 0). This offset is permanent and cumulative. |
z | Number (World Coords) |
The offset along the Z axis by which the object is moved relative to its drawing origin (0, 0). This offset is permanent and cumulative. |
trans | Array(3) [x, y, z] |
The offset along the X, Y, and Z axes in that order stored in a 3 element array. The object is moved relative to its drawing origin's current position. This offset is permanent and cumulative. |
rot | Array(4) [vx, vy, vz, degs] |
The first 3 elements of the array argument define a vector (vx, vy, vz). The fourth element represent the number of degrees of rotation about the direction vector. This rotation is centered on the world coordinate origin. This rotation is permanent and cumulative. |
rotX | Number (deg) | The angle by which the object is rotated about the X axis. This is a permanent rotation not reset after rendering. The effect of the rotation will depend on whether a translate has been inserted prior to the xRot. This rotation is permanent and cumulative. |
rotY | Number (deg) | The angle by which the object is rotated about the Y axis. This is a permanent rotation not reset after rendering. The effect of the rotation will depend on whether a translate has been inserted prior to the yRot. This rotation is permanent and cumulative. |
rotZ | Number (deg) | The angle by which the object is rotated about the Z axis. This is a permanent rotation not reset after rendering. The effect of the rotation will depend on whether a translate has been inserted prior to the zRot. This rotation is permanent and cumulative. |
scl | Number | The scaling factor by which the objects dimensions are scaled, the expansion or contraction is centered on the world coordinate origin (0,0,0). This is a permanent change in size not reset after rendering. This scaling is permanent and cumulative. |
sclNonUniform | Array(3) [sclX,sclY,sclZ] |
The independent scale factors in each axis direction by which the objects dimensions are scaled. The expansion or contraction is centered on the world coordinate origin (0,0,0). This is a permanent change in size not reset after rendering. The effect of the scaling will depend on whether a translate has been inserted prior to the scl. This scaling is permanent and cumulative. |
Path3D and Shape3D methods
Construction methods
Syntax:
obj.setProperty(propertyName, val);
Description:
Sets the value for the property specified by the string 'propertyName'. The value must be of an appropriate type and fall within the useful range of values for the property.
Parameters:
propertyName: String- The name of the Path3D property the value of which is to be set. It must be one of the strings listed in column 1 of the Path3D and Shape3D properties tables. The string value is not case sensitive.
value: (various types) - The new value for the property.
Syntax:
const newObj = obj.dup();
Description:
dup creates a new object of the same type as 'obj' and copies all the properties and methods from the original into the new object. The properties are copies of the values not references to the original. This method is useful a useful shortcut when creating complex objects made from similar components.
Parameters:
none.
Returns:
newObj: A new object which is a duplicate of the original.
Syntax:
obj.flipNormal();
Description:
If a Path3D previously defined and has the calculated normal the wrong way, so the back surface is shown in a default color instead of the correct colored front, then the Path3D method flipNormal called on the object will flip the normal to the opposite direction.
Parameters:
None
Pointer event methods
Syntax:
obj.enableDrag(dragCallback [, grabCallback [, dropCallback]]);
Description:
This method takes as parameters the user defined functions to be called mousedown, mousemove or mouseup (or mouseout) events. It saves a reference to 'obj' in the array of objects to be checked when a mousedown event occurs on the canvas.
Once an object is enabled, the Cango3D 'mousedown' event handler will check if the mouse event occurred within the outline of the object. If it did then for all subsequent 'mousemove' events the user 'dragCallback' function is called, and the updated event data object will be passed as its only argument. See Event Data properties for details of the event data properties. If the user code provides a 'grabCallback' function it will be called when the 'mousedown' event occurs within the object outline. If the user code provides a 'dropCallback' function it will be called for any subsequent 'mouseup' or 'mouseout' event. Drag-n-drop callbacks are executed in the scope of the Cango3D context that rendered the object.
Parameters:
dragCallback: Function - This function to be called when a 'mousemove' event occurs after the mousedown event has occurred within the outline of 'obj'. An event data object containing various data for use by event handlers is the only parameter passed when the function is called.
grabCallback: Function (optional) - This function to be called when a 'mousedown' event occurs within the outline of 'obj'. An event data object containing various data for use by event handlers is the only parameter passed.
dropCallback: Function (optional) - This function to be called when a mouseup or mouseout event occurs after the mouse down event. An event data object containing various data for use by event handlers is the only parameter passed.
Syntax:
obj.disableDrag();
Description:
Sets the obj.dragNdrop property to 'null' and removes the reference to obj from the array of objects to be checked for a hit on mousedown events.
Parameters:
none
Syntax:
obj.enableClick(clickCallback);
Description:
This method takes as parameters the user defined functions to be called on a 'click' event. It saves a reference to 'obj' in the array of objects to be checked when a click event occurs on the canvas.
Once an object is enabled, any time a 'click' event (a mousedown followed by a mouseup event within the outline of the object) occurs the user 'clickCallback' function will be called. This callback function receives the updated event data object. See Event Data properties for details of the event data properties. Drag-n-drop callbacks are executed in the scope of the Cango3D context that rendered the object.
Parameters:
clickCallback: Function - This function to be called when a 'click' event occurs within the outline of 'obj'. An event data object containing various data for use by event handlers is the only parameter passed.
Syntax:
obj.disableClick();
Description:
Sets the obj.dragNdrop property to 'null' and removes the reference to obj from the array of objects to be checked for a hit on click events.
Parameters:
none
Syntax:
obj.transformRestore();
Description:
After an object is rendered to the canvas the array of inherited dynamic transforms that have been applied is cleared. Hence to redraw an object in the same position and orientation requires the transforms to be reapplied. The transformRestore method is available to simplify the code to do this. Applying the transformRestore applies a minimal set of transforms that result in the the same orientation, size and position that the full set of transforms applied to the object as it was most recently rendered onto canvas.
This method is useful in drag and drop handlers where objects that are not being dragged can be simply redrawn and appear unchanged and the target object being dragged can be correctly positioned using the incremental movement of the pointer.
Parameters:
none
Pointer event callback EventData properties
Property | Type | Description |
---|---|---|
target | Group3D or Path3D | The object actually being dragged, the Group3D or Path3D whose 'enableDrag' method was called. |
pointerPos | Object {x, y, z} | Object with x, y and z properties holding the coordinates of the cursor in world coordinates at the time of the event. |
dragPos | Object {x, y, z} | Object with x, y and z properties holding the current distances in world coordinates that the pointer has move since the grab event in the direction of the X,Y and Z world coordinate axes directions respectively. |
dragOfs | Object {x, y, z} | Object with x, y and z properties holding the difference in new pointer position from the position when the target object was last drawn (ie. when the event handler was last called). Distances are in world coordinates values. |
dragAngles | Object {xa, ya, za} | 'dragAngles.xa' is the angle of rotation about the world coordinate X axis generated by the vertical movement (dragPos.y) of the pointer from its position at the grab event. The angle is calculated as the angle swept by a 50px arm. Similarly the 'dragAngles.ya' is the rotation about the Y axis generated by the horizontal movement (dragPos.x). 'dragAngles.za' is the angle of rotation about the Z axis generated by the tangential movement of the pointer about the Z axis (ie. arctan(dY/dX)). All angles are measured in degrees. |
dragOfsAngles | Object {xa, ya, za} | 'dragOfsAngles.xa' is the increment of rotation about the X axis generated by the movement of the pointer from its position when the target object was last rendered ie. the difference between the current dragAngles.xa and the saved values when the event handler was last called. The 'dragOfsAngles.ya' and dragOfsAngles.ya' and 'dragOfsAngles.za' are the corresponding incremental change in the values and their respective dragAngles. All angles are measured in degrees. |
Group3D
Group3D can be created using the Group3D constructor. The objects to be grouped are passed as parameters. Objects can be added later using the Group3D.addObj method.
The Group3D object has the property 'children', an array containing any Path3D, Shape3D or Group3D or any Cango3D Extension object such as Panel3D, Text3D, ObjectByExtrusion3D etc. Objects are added by passing individual objects or arrays of objects to the Group3D constructor. Objects may also be added to the Group3D by the addObj method which can similarly take individual objects, Group3Ds or arrays of them as parameters. Children can be removed by the deleteObj method.
Group3D also have several transform methods that will be inherited by all their children recursively: grp.translate, grp.rotate and grp.scale etc.
All the children of a Group3D can be 'drag-n-drop' enabled or disabled with a call to the Group3D methods, grp.enableDrag and grp.disableDrag.
Syntax:
const obj = new Group2D(...);
Description:
Creates a Group3D object with a 'children' array property which hold Path3D and other Group3D. The Group3D object has methods to apply transforms to children, and recursively apply them to the children of child Group3D. The objects forming the Group3D's children may be passed as parameters, either individual Path3D or Group3D or arrays of either. More objects can be added to the group after creation, by using the addObj method.
Parameters:
. . . : Path3D, Shape3D, Group3D, Extension Objects or arrays of them - The 'arguments' property is parsed to flatten nested arrays and extract all objects which are then are added to the grp.children array.
Group3D methods
Construction methods
Syntax:
grp.addObj(...);
Description:
The method adds Path3D or Group3D to the Group3D 'grp'. The arguments can be single Path3D or Group3D or arrays of either. The array structure is discarded and the individual Path3D or Group3D are added to the grp.children array.
Parameters:
. . . : Path3D, Shape3D, Group3D, Extension Objects or arrays of them - The 'arguments' property is parsed to flatten nested arrays and extract all objects which are then are added to the grp.children array.
Syntax:
grp.deleteObj(obj);
Description:
If 'obj' is a child of the Group3D 'grp' then it is removed from the array of children.
Parameters:
obj: Path3D or Group3D - The child to be removed from the Group3D.
Pointer event methods
Syntax:
grp.enableDrag(dragCallback, grabCallback, dropCallback);
Description:
Calls the Path3D.enableDrag method on all grp's Path3D children, and also calls the method on all the Path3D children of grp's Group3D children and so on recursively. The user defined callback function 'dragCallback' and the optional 'grabCallback', 'dropCallback' are passed to the Path3D.enableDrag method.
To simplify writing grab, drag and drop functions, the callbacks are passed an event data object which has properties useful to handlers. These properties are listed in the Event Data properties section below.
Parameters:
dragCallback: Function - This function to be called when a 'mousemove' event occurs after the mousedown event has occurred with the outline of the grp's descendent Path3D. An object containing the current event data is the only parameter passed when the function is called.
grabCallback: Function (optional) - This function to be called when a 'mousedown' event occurs within the outline of the grp's descendent Path3D. An object containing the current event data is the only parameter passed when the function is called.
dropCallback: Function (optional) - This function to be called when a mouseup or mouseout event occurs after the mousedown event. An object containing the current event data is the only parameter passed when the function is called.
Syntax:
grp.disableDrag();
Description:
Sets the grp.dragNdrop property to 'null' and removes the reference to grp's children from the array of objects to be checked for a hit on mousedown events.
Parameters:
none
Syntax:
grp.enableClick(clickCallback);
Description:
Calls the Path3D.enableDrag method on all grp's Path3D children, and also calls the method on all the Path3D children of grp's Group3D children and so on recursively. The user defined callback function 'clickCallback' is passed to the Path3D.enableClick method.
To simplify writing click callback functions, the callback is passed an event data object containing properties useful in the event handler. These are listed in the Event Data properties section below.
Parameters:
clickCallback: Function - This function to be called when a 'click' event occurs within the outline of the grp's descendent Path3D. An object containing the event data is the only parameter passed when the function is called.
Syntax:
grp.disableClick();
Description:
Sets the grp.dragNdrop property to 'null' and removes the reference to grp's children from the array of objects to be checked for a hit on mousedown events.
Parameters:
none
Hard transform methods
Syntax:
grp.setProperty(propertyName, value);
Description:
Calls the Path3D.setProperty method on all grp's Path3D children, and also calls the method on all the Path3D children of grp's Group3D children and so on recursively. The 'propertyName' and 'value' arguments are passed as is to the Path3D method.
Parameters:
propertyName: String- The name of the Path3D property the value of which is to be set. It must be one of the strings listed in column 1 of the Path3D and Shape3D properties tables. The string value is not case sensitive.
value: (various types) - The new value for the property.
Soft transform methods
Syntax:
obj.rotate(vx, vy, vz, degs);
Description:
Adds a transform object is created to rotate the Group3D children by 'degs' degrees about the axis defined by a vector starting at the world coordinate origin (0,0,0) to the point (vx, vy, vz). The transform object is inherited by the children of the Group3D and by the children of any Group3D children recursively. Transforms are applied in order of insertion. The transform is not permanent as the Group3D's array of accumulated transforms is cleared after rendering.
Parameters:
vx, vy, vz: Numbers - The x, y, and z components of a vector from the origin to the point vx,vy,vz. The vector can be of any length (it will be converted to unit length before use). The vector defines the axis, about which all points of the object will be rotated. If the rotate is applied before any translate, the axis of rotation will appear to be about the drawing origin rather than the world coordinate origin.
degs: Number - the angle by which this transform will rotate an object. The angle 'degs' is measured in degrees, +ve anti-clockwise if the axis vector is pointing out of the screen (right hand rule).
Syntax:
obj.rotateX(degs);
Description:
A transform object is created to rotate the Group3D children by 'degs' degrees about the X axis when next rendered. The transform object is inherited by the children of the Group3D and by the children of any Group3D children recursively. Transforms are applied in order of insertion. The transform is not permanent as the Group3D's array of accumulated transforms is cleared after rendering.
Parameters:
degs: Number - the angle by which this transform will rotate the group. The angle 'degs' is measured in degrees, +ve anti-clockwise looking along the X axis toward the origin (right hand rule).
Syntax:
obj.rotateY(degs);
Description:
A transform object is created to rotate the Group3D children by 'degs' degrees about the Y axis when next rendered. The transform object is inherited by the children of the Group3D and by the children of any Group3D children recursively. Transforms are applied in order of insertion. The transform is not permanent as the Group3D's array of accumulated transforms is cleared after rendering.
Parameters:
degs: Number - the angle by which this transform will rotate the group. The angle 'degs' is measured in degrees, +ve anti-clockwise looking along the Y axis toward the origin (right hand rule).
Syntax:
obj.rotateZ(degs);
Description:
A transform object is created to rotate the Group3D children by 'degs' degrees about the Z axis when next rendered. The transform object is inherited by the children of the Group3D and by the children of any Group3D children recursively. Transforms are applied in order of insertion. The transform is not permanent as the Group3D's array of accumulated transforms is cleared after rendering.
Parameters:
degs: Number - the angle by which this transform will rotate the group. The angle 'degs' is measured in degrees, +ve anti-clockwise with the Z axis pointing out of the screen (right hand rule).
Syntax:
grp.roll(degs);
Description:
Adds a rotation to the transform matrix to be applied to the Group3D's descendent objects when rendered. The object will be rotated by 'degs' degrees about its internal X axis. This is the X axis that the object's coordinates were referenced to at the time of construction. This transform naming convention follows the yaw, pitch, roll terms used in the aerospace industry (X axis is in the direction of flight, +ve roll lifts the left wing). The yaw, pitch and roll transforms are applied to the object before any other transform. The transform is cleared after rendering.
Parameters:
degs: Number - the angle by which this transform will rotate an object. The angle 'degs' is measured in degrees, +ve clockwise looking along the X axis away from the origin.
Syntax:
grp.pitch(degs);
Description:
Adds a rotation to the matrix to be applied to the Group3D's descendent objects when rendered. The object will be rotated by 'degs' degrees about its internal Y axis. This is the Y axis that the object's coordinates were referenced to at the time of construction. This transform naming convention follows the yaw, pitch, roll terms used in the aerospace industry (Y axis is to the right of the direction of flight, +ve pitch angle lifts the nose). The yaw, pitch and roll transforms are applied to the object before any other transform. The transform is cleared after rendering.
Parameters:
degs: Number - the angle by which this transform will rotate an object. The angle 'degs' is measured in degrees, +ve clockwise looking along the Y axis away from the origin.
Syntax:
grp.yaw(degs);
Description:
Adds a rotation to the matrix to be applied to the Group3D's descendent objects when rendered. The group will be rotated by 'degs' degrees about its internal Z axis. This is the Z axis that the Groups's coordinates were referenced to at the time of construction. This transform naming convention follows the yaw, pitch, roll terms used in the aerospace industry (Z axis is down, +ve yaw will turn to the right). The yaw, pitch and roll transforms are applied applied to the object before other transforms. The transform is cleared after rendering.
Parameters:
degs: Number - the angle by which this transform will rotate an object. The angle 'degs' is measured in degrees, +ve clockwise looking along the Z axis away from the origin.
Syntax:
obj.scale(scl);
Description:
A transform object is created to scale the Group3D children by 'scl' when next rendered. The objects dimensions enlarge or contract centered on the world coordinate drawing origin. The transform object is inherited by the children of the Group3D and to the children of any Group3D children recursively. Transforms are applied in order of insertion. The transform is not permanent as the Group3D's array of accumulated transforms is cleared after rendering.
Parameters:
scl: Number - A number, greater than 0, by which the objects dimensions relative to it's drawing origin are scaled.
Syntax:
obj.scaleNonUniform(sclX, sclY, sclZ);
Description:
A transform object is created to scale the Group3D children when next rendered. Each child Path3D's coordinates will be scaled by a factor 'sclX' in the X axis direction and by sclY and sclZ in the Y and Z axis directions respectively. The object's dimensions enlarge or contract centered on the world coordinate drawing origin. The transform object is inherited by the children of the Group3D and to the children of any Group3D children recursively. Transforms are applied in order of insertion. The transform is not permanent as the Group3D's array of accumulated transforms is cleared after rendering.
Parameters:
sclX: Number - value, greater than 0, by which the objects X coordinates are scaled relative to it's drawing origin.
sclY: Number - value, greater than 0, by which the objects Y coordinates are scaled relative to it's drawing origin.
sclZ: Number - value, greater than 0, by which the objects coordinates are scaled relative to it's drawing origin.
Syntax:
obj.translate(x, y, z);
Description:
A transform object is created to translate the Group3D children when next rendered. The transform is applied to all the children of the Group3D and to the children of any Group3D children recursively. Each Path3D's drawing origin will be offset from its current location by x, y and z world coordinate units in the X direction, Y direction and Z direction respectively. Transforms are applied in order of insertion. The transform is not permanent as the Group3D's array of accumulated transforms is cleared after rendering.
Parameters:
x, y, z: Numbers - The distance in the X, Y and Z directions respectively that the object will be moved relative to current position which will be the world coordinate origin unless a prior translate transform has been applied.
Syntax:
grp.transformRestore();
Description:
When a group of objects is rendered to the canvas the array of transforms applied is cleared. Hence to redraw objects in the same position and orientation requires the transforms to be reapplied. The transformRestore method is available to simplify the code to do this, it applies a minimal set of transforms equivalent to those dynamic transforms applied when it was most recently rendered onto canvas.
This method is useful in drag and drop handlers or animations where the motion is defined in increments of the pointer movement or the calculated frame-to-frame position difference. For elements within the scene but not moved this method can be used to have these objects redrawn and appear unchanged.
Parameters:
none
Rotation axes
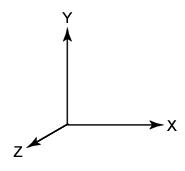
When an Object is constructed all coordinates are referenced to the world coordinates, X axis to the right, Y axis up the screen and Z axis out of the screen. The object can have Transform Properties applied that are permanent, essentially part of the construction. Rotation transform properties are reference to the world coordinate axes.

Objects have dynamic or soft transform methods which apply transforms to the object's coordinates prior to rendering after which the array of transforms to be applied is cleared. These transforms are suitable for drag-n-drop and animation actions. Dynamic or soft rotation transforms. The direction of rotation follows the right hand rule where positive rotation angle rotates the object clockwise when looking along the axis away from the origin.

The roll, pitch and yaw rotation directions follow the right hand rule and the axes that they rotate about are in the directions of the world coordinate axes relative to the object as they were at object construction including the effects of any transform properties assign to the object.
Cango3D Extension Objects
Cango3D extension objects are Group3D constructor methods that return 3D objects that are composites of the basic Path3D and Shape3D. They support all the methods and properties of a Group3D. They are distinguished by supporting preSort and preRender methods. The preSort method provides a way to make modifications to transforms and properties of the object just before they are sorted in the oder to rendered modifications can alter the order of rendering and hence which objects overlay others. If a 'preRender' method is provide it will be applied immediately before rendering so modifications to the array of transforms and other object properties can be applied.
Panel3D
The Panel3D is a Group3D sub-class that behaves just as a Shape3D but the Panel3D is constructed from 2D SVG commands with all coordinates lying in the XY plane. Additionally it has the 'label' method which attaches a Text3D object that will then behave as part of the Panel3D.
Syntax:
const pnl = new Panel3D(descriptor, options);
Description:
A 3D plane shape defined by a set of 2D SVG style commands with all coordinates in the XY plane. Once instantiated it behaves just as a Shape3D object. When rendered a Panel3D outline is filled with color value of its 'fillColor' property if it is facing toward the observer, or 'backColor' if facing away.
Parameters:
descriptor: Array, String or PathSVG object - The array or string data defines a 2D path in the XY plane defining the Panel3D's outline. The elements are single letter SVG path commands and their associated 2D coordinates. The full set of SVG path commands is supported. The outline path data is often imported in 2D SVG format, copied as a string from an SVG editor such as InkScape or as a PathSVG object which has static methods defining commonly used 2D shapes; circle, ellipse, square, rectangle and triangle and methods to modify SVG data to create novel paths.
options: Object - The key-values pairs define the values of the various properties of the Shape3D the supported keys are listed in the Path3D and Shape3D properties tables.
Panel3D additional methods
As a subclass of the Group3D, Panel3D inherits all the Group3D methods in addition it has the 'label' and 'dup' methods:
Syntax:
pnl.label(textObj, xOfs, yOfs);
Description:
This method allows a Panel3D to attach a Text3D object forming a Group3D so that the panel and the text label will always be drawn together and the label will always be rendered in front of the panel face.
Multiple labels may be added to a Panel3D.
Parameters:
txt: Text3D - The text label to be drawn at the same location as the Panel3D and always in front.
xOfs, yOfs: Numbers - (optional) X and Y offsets of the Text3D object's drawing origin from the drawing origin of the Panel3D.
const pnlCopy = pnl.dup();
Description:
This method returns a Panel3D which is a duplicate of the calling Panel3D. If the Panel3D has had a label applied then the label will be copied too.
Parameters:
none
Text3D
The Text3D Group3D sub-class that behaves just as a Path3D object but the Text3D descriptor is a string. Each character of the string is converted to multi-segment Path3D object and added to the Text3D group.
Syntax:
const txt = new Text3D(str, options);
Parameters:
str: String - The string of characters to be converted to 3D objects and written to the canvas.
options: Object - The key-values pairs define the values of the various properties of the Text3D. The supported keys are listed in the Path3D and Shape3D properties tables. In addition there are three Text3D specific properties too:
Additional properties
Property | Type | Description |
---|---|---|
fontSize | Number | Font size in Pixels (default 15px) |
fontWeight | Number | Font weight 100..900 (default 400) |
lorg | Number [1,2,3,4,5,6,7,8,9] | Specifies the location of the drawing origin within the bounding rectangle of text. lorg=1 locates the drawing origin at the top,left of the text, lorg=2 locates it at the top,center of the text, and so on to lorg=9 which locates the drawing origin at the lower right corner. Default lorg=1. In the figure below the intersection of the Text3D labels are examples of the 9 possible lorg values. ![]() |
Text3D additional method:
As a subclass of the Group3D, Text3D inherits all the Group3D methods in addition it has the 'dup' method:
Syntax:
const pnlCopy = pnl.dup();
Description:
This method returns a Text3D which is a duplicate of the calling Text3D.
Parameters:
none
Sphere3D
The Sphere3D is a pseudo 3D object actually a 2D circular object that has shading and specular highlight to simulate a sphere.
Syntax:
const orb = new Sphere3D(diameter, options);
Description:
Creates a pseudo 3D sphere. It is actually a disc with shading to simulate a sphere. The disc is always drawn face toward the observer.
Parameters:
diameter: Number - The diameter of the sphere measured in world coordinates.
options: Object - The key-values pairs define the various properties of the Sphere3D. The Sphere3D supports the set of Shape3D properties listed in the Path3D and Shape3D properties tables. The Sphere3D supports two additional properties:
Additional properties
Property | Type | Description |
---|---|---|
shine | Number 0 .. 1 | Specifies the relative shine of the sphere. This sets the brightness of the specular reflection of the light source. 1 is shiny as a billiard ball, 0 is flat color with no specular reflection. Default value is 0.5. |
ambient | Number 0 .. 1 | Sets the relative brightness of the dark side of the sphere. A value of 1 has the dark side as bright as the front, a value 0 has the dark side black. Default value is 0.7. |
ObjectByRevolution3D
The ObjectByRevolution3D is sub-class of Group3D that behaves as a group of Shape3D objects constructed by revolving the descriptor profile about the Y axis forming an object like the segments of an orange.
ObjectByRevolution3D constructor
Syntax:
const obj = new ObjectByRevolution3D(svgData, segments, straight, options);
Description:
The path defined by the 'svgData' is revolved 360° about the Y axis in 'segments' number of steps. At each step a Shape3D object is formed from the pairs of paths forming the sides of the panel and top and bottom are joined by circular arcs (by default) or straight lines if the 'straight' parameter evaluates to 'true'. The array of panels forming the object are added to a Group3D object which is returned.
Parameters:
svgData: Array, String or PathSVG object - An array or string containing in SVG (2D) format consisting of single letter SVG drawing commands followed by x,y coordinates. The first command must be 'M' followed by a coordinates specifying the x,y values of the starting point of the path. This is followed by commands specifying line segments. The path should be simple starting from the top of the profile (maximum Y coordinate) and monotonically decreasing to the bottom, all points should have positive X coordinate values.
segments: Number - Specifies the number of segments forming the object. The angle subtended by each segment will be 360/segments. The object is created like segments of an orange. The larger the number of segments the smoother the color gradations will be on the final shape.
straight: Boolean - An optional boolean, if true the sections of adjacent profiles are joined by straight lines if false or undefined the segments are joined by circular arcs.
options: Object - an object whose key-value pairs set initial values for the various properties on the object's panels. All the options supported by Shape3D are supported are shown in the Path3D and Shape3D properties table.
Example:
The figure below shows a graph of the SVG path on the left and the 3D rotated object on the right by creating the ObjectByRevolution3D with this code snippet:
... const glass = "M 118,0 c 0,-5.71 -0.25,-11.05 -1.89,-15.69 -1.64,-4.63 -4.69,-8.53 -10.24,-11.44 -21.68,-11.40 -52.93,-20.24 -68.17,-26.25 -7.61,-3 -14,-6.24 -19,-11.87 -5,-5.62 -8.61,-13.63 -10.66,-26.16 -1.63,-10 -3.85,-75.22 1.42,-82.85 3.45,-4.98 5.68,-8 29.64,-13.85 10,-2.41 19.63,-3.28 22.49,-6.85"; const glassObj = new ObjectByRevolution3D(glass, 24, false, {fillColor:"yellow", backColor:"lightGreen"}); ...
ObjectByExtrusion3D
The ObjectByExtrusion3D is sub-class of the Group3D that behaves like a multi sided object, each side a Shape3D and closed at both ends by matching Panel3D. Each side facet is a Shape3D constructed from a segment of the outline path of front end panel joined to the matching segment of the back end panel joined by straight lines.
ObjectByExtrusion3D constructor
Syntax:
const obj = new ObjectByExtrusion3D(svgData, len, options);
Description:
Generates a Group3D holding an array of Shape3D representing a multi-panel 3D object formed by extruding the outline by the 'svgData' parameter for a distance specified by 'len'. The object's top is a Panel3D formed from the svgData outline, the bottom is a duplicate of the top shifted a distance 'len' along the -ve Z axis. The bottom's normal is flipped to point outward from the object. The number of panels forming the walls of the object will be determined by the number of segments in the svgData path. For each segment a Shape3D is formed from a segment in the top and the corresponding segment in the bottom joined by straight line segments. The set of Shape3Ds along with the top and bottom Panel3Ds are added to a Group3D object which is returned.
Parameters:
svgData: Array, String or PathSVG object - An array or string containing in SVG (2D) format consisting of single letter SVG drawing commands followed by x,y coordinate pairs defining the outline path to be extruded.
len:Number - The distance in world coordinates by which the outline will be extruded to form the surface.
options: Object - holds key-value pairs to set initial values for the various properties on the object's panels. All the Shape3D optional properties are supported the keys are shown in the Path3D and Shape3D properties tables.
Example:
The figure below shows a graph of the SVG path on the left and the 3D extruded object on the right by calling the ObjectByExtrusion3D constructor with this code snippet:
... const depth = 200; const pentagon = ["M",0,scl, "L",-s1,c1, -s2,-c2, s2,-c2, s1,c1, "Z"]; const pentObj = new ObjectByExtrusion3D(pentagon, depth, {fillColor:"yellow"}); ...
SurfaceByExtrusion3D
The SurfaceByExtrusion3D is sub-class of Group3D that behaves as a multi sided object, each side a Shape3D constructed a segment of the outline SVG path data and joined to the matching segment of a copy of the path separated by the requested length.
SurfaceByExtrusion3D constructor
Syntax:
const obj = new SurfaceByExtrusion3D(svgData, len, options);
Description:
Generates a Group3D holding an array of Path3D representing a multi-panel 3D object formed by extruding the outline by the 'svgData' parameter for a distance specified by 'len'. The top edge of the surface is defined by the 'svgData' outline converted to a Cgo3D format, the bottom is a duplicate of the top translated a distance 'len' along the -ve Z axis. The number of panels forming the surface object will be determined by the number of segments in the svgData path. For each segment a Shape3D is formed from a segment in the top edge and the corresponding segment in the bottom edge joined by straight line segments. The set of Shape3Ds forming the surface is added to a Group3D object which is returned.
Parameters:
svgData: Array, String or PathSVG object - An array or string containing in SVG (2D) format consisting of single letter SVG drawing commands followed by x,y coordinate pairs defining the outline path to be extruded.
len: Number - The distance in world coordinates by which the outline will be extruded to form the surface.
options: Object - holds key-value pairs to set initial values for the various properties on the object's panels. All the options supported by Shape3D are supported, the full list is shown in Path3D and Shape3D properties tables.
Example:
The figure below shows a graph of the SVG path on the left and the 3D extruded surface on the right by calling the SurfaceByExtrusion3D constructor with this code snippet:
... const depth = 200; const glassProfile = "M 118,0 c 0,-5.71 -0.25,-11.05 -1.89,-15.69 -1.64,-4.63 -4.69,-8.53 -10.24,-11.44 -21.68,-11.40 -52.93,-20.24 -68.17,-26.25 -7.61,-3 -14,-6.24 -19,-11.87 -5,-5.62 -8.61,-13.63 -10.66,-26.16 -1.63,-10 -3.85,-75.22 1.42,-82.85 3.45,-4.98 5.68,-8 29.64,-13.85 10,-2.41 19.63,-3.28 22.49,-6.85"; const glass = new SurfaceByExtrusion3D(glassProfile, depth, {fillColor:"yellow", backColor:"lightGreen"}); ...
Global utility methods
A few functions that may be of use in in writing apps using Cango3D graphics have been made available as globals.
Syntax:
const degs = calcIncAngle(p1, p2, p3);
Description:
Calculates the included angle between the vectors from p1 to p2 and p1 to p3. If A is the vector defined as p2 - p1, and B is the vector p3 - p1, then the included angle (less than 180°) between A and B will be returned. The sign may be determined from the cross product A×B direction (determined from right hand rule). The direction of the positive angle is counter-clockwise when the cross-product is pointing at you.
Parameters:
p1: Object {x:, y: z:} - A point in 3D space which is taken as the base point of the two vectors whose included angle is to be measured.
p2y: Object {x:, y: z:} - A point in 3D coordinates, the vector from p1 to p2 defines the first vector A.
p3: Object {x:, y: z:} - A point in 3D coordinates, the vector from p1 to p3 defines the second vector B.
Returns:
Number - An angle between the two 3D vectors measured in degrees.
Syntax:
const norm = calcNormal(p1, p2, p3);
Description:
Calculates the unit vector perpendicular to the plane defined by the points p1, p2 and p3. If A is the vector from p1 to p2 (p2 - p1), and B is the vector from p1 to p3 (p3 - p1), then the cross product AxB is calculated and normalised to have unit length. The direction of the normal will be given by the 'right hand rule', if A were along the X axis, and B along the Y axis the normal would be along the Z axis.
Parameters:
p1: Object {x:, y: z:} - A point in 3D space which is taken as the base point of the two vectors defining the plane.
p2: Object {x:, y: z:} - A point in 3D coordinates, the vector from p1 to p2 defines a line in the plane.
p3: Object {x:, y: z:} - A point in 3D coordinates, the vector from p1 to p3 defines a second line in the plane.
Returns:
Object {x:, y: z:} - A point in 3D space unit distance (world coordinates) from the origin in the direction normal to the plane defined by the three points p1, p2 and p3.
Syntax:
const dataAry = svgToCgo3D(svgData, xRef, yRef);
Description:
This is utility accepts 2D path data in SVG path syntax and returns 3D path data in Cgo3D format with all Z coordinate values set to 0. SVG data is passed as a String or as an Array.
The SVG string format is designed to handle path definitions imported, via copy and paste, from SVG source editors. The path to be copied can be easily identified within the SVG source code. The path's 'd' parameter holds the command letters and 2D coordinates for each segment of the path. The first command must be 'M' (move to) followed by a coordinates specifying the x,y values of the starting point of the path. This is followed by an arbitrarily long set of commands and associated coordinate values such as 'L' for a line segment, 'C' for a cubic Bézier curve, etc. All the SVG syntax commands are supported 'A','S','T', etc. commands are converted to simple cubic Bézier curves which preserve their shape under 3D transformation. The coordinates following each command letter are all in world coordinates.
Since all SVG editors use a coordinate system with Y coordinates +ve down the screen, 'svgData' passed as a will be assumed to be in this format and svgToCgo3D will flip all the Y coordinates to match the Cango3D Right Handed coordinate system.
The 'xRef,yRef' parameters are available to shift the drawing origin of the coordinates. The SVG editors usually have the drawing origin offset away in the top corner, 'xRef,yRef' allow it to be shifted to a more convenient position.
The 'svgData' may also be in an array containing single letter SVG commands followed by their corresponding 2D coordinates. The coordinate x,y pairs are numbers not strings. They are assumed to already be in Right Handed Cartesian coordinate system with Y +ve up the screen. This format is suitable for programmatically generated data.
Parameters:
svgData: String, Array or PathSVG - A string or array or PathSVG object holding the drawing instructions for the path. If 'svgData' is a string then it is assumed be in Left Handed Cartesian format used for SVG path data. If 'svgData' is an Array or PathSVG it is assumed to be in RHC format.
xRef, yRef: Numbers - X and Y offsets to be added to all the drawing coordinates of string data, to effectively move the path's drawing origin, measured in the original SVG editor coordinates (which will subsequently be interpreted as world coordinates).
Returns:
A Cgo3D format data array consisting of single letter commands and their numeric coordinate x,y,z triplets (all z values will initially be 0) suitable for input to the Cango3D 'compile' methods.
Cgo3Dsegs
The Cgo3Dsegs is a sub-class of the Array object whose constructor takes an array of Cgo3D commands and coordinates defining a path and returns an array of path segments and provides methods to transform and modify the path. These methods include translate, rotate, scale and scaleNonUniform methods along with methods dup, joinPath, appendPath and revWinding. These methods return a new Cgo3Dsegs object so that the methods can be chained in functional programming style.
To create a 'Cgo3Dsegs' object you must first create an array of Cgo3D commands which are SVG style commands but restricted to only 'M', 'L', 'C' and 'Z' and their associated absolute coordinate triplets, is the style:
['M',x,y,z, 'L',x,y,z, ... 'C',c1x,c1y,c1z,c2x,c2y,c2z,x,y,z, ... 'Z']
If the path is defined by a string of SVG (2D) commands then the svgToCgo3D utility function may be used to convert the string to the equivalent Cgo3D array suitable to pass to the Cgo3Dsegs constructor.
Cgo3Dsegs constructor
Syntax:
const pathData = new Cgo3Dsegs(data);
Description:
This constructor converts the input Cgo3D commands and associated parameters in 'data' into a sequence of path segments. An array is returned each element of which is an object defining a single segment of the path.
Parameters:
data: Array of Cgo3D data - an array holding a series of commands and their associated 3D parameters. The restricted set ("M", "L", "C", "Z") of SVG commands is supported.
Returns:
Cgo3Dsegs object - each element of the Cgo3Dsegs array returned defines a segment of the path. The returned object is suitable for use as the descriptor of an Shape3D or Path3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Example
The examples create Cgo3Dsegs objects.
const path1 = new Cgo3Dsegs(["M",8.24,-0.1,0, "L",1.2,0.2,0, 1.6,-1.2,0.2, "C",8.3,8.3,0, 0.5,1,4.9, 6.6,3,9.6, "Z"]); const path2 = new Cgo3Dsegs(svgToCgo3D("M 3,1, l -1.2,0.2 0,1.6 -1.2,0.2 A 8.3,0,5,0,0,1,4.9,6.6")); const path3 = path1.joinPath(path2) .scale(7.5);
Cgo3Dsegs Methods
Syntax:
path1.appendPath(path2);
Description:
The appendPath method extends path1 segments array by appending the path2 segments. Since the path2 commands must start with a 'M' command or 'moveTo' the resulting path has a separate, non contiguous section added.
Parameters:
path2: Cgo3Dsegs object or Cgo3D data array - The Cgo3D path data defining a path to be appended to path1.
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
const newObj = obj.dup();
Description:
The dup method creates a new Cgo3Dsegs array object, copying the segments array and all the methods from the original into the new object.
Parameters:
none.
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
pathSegs.joinPath(extPath);
Description:
The joinPath method extends pathSegs array by adding the extPath segments. If pathSegs is closed ie. the last segment is a 'closePath' command then the closePath segment is deleted. The initial 'M' command or 'moveTo' segment of the extPath is deleted so that the resulting path is continuous and extended.
Parameters:
extPath: Cgo3Dsegs Array or String of Cgo3D data - The array of data defining a path to be joined to pathSegs.
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
const newObj = obj.revWinding();
Description:
The revWinding method creates a Cgo3Dsegs array object segments represent the same path as the original but the path segments are rearranged in reverse order. This method is useful when creating an objects made from a predefined path but which would have its normal facing the wrong way. Traversing the outline in the reverse direction flips the normal.
Parameters:
none.
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
obj.rotateX(degs);
Description:
Rotates the path segment coordinates by 'degs' degrees about the X axis.
Parameters:
degs: Number - the angle by which this method will rotate the path segments. The angle 'degs' is measured in degrees with rotation direction anti-clockwise looking along the X axis toward the origin ('right hand grip' rule).
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
obj.rotateY(degs);
Description:
Rotates the path segment coordinates by 'degs' degrees about the Y axis.
Parameters:
degs: Number - the angle by which this method will rotate the path segments. The angle 'degs' is measured in degrees with rotation direction anti-clockwise looking along the Y axis toward the origin ('right hand grip' rule).
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
obj.rotateZ(degs);
Description:
Rotates the path segment coordinates by 'degs' degrees about the Z axis.
Parameters:
degs: Number - the angle by which this method will rotate the path segments. The angle 'degs' is measured in degrees with rotation direction anti-clockwise looking along the Z axis toward the origin ('right hand grip' rule).
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
obj.rotate(vx, vy, vz, degs);
Description:
Rotates the path segment coordinates by 'degs' degrees about the axis defined by a vector starting at the world coordinate origin (0,0,0) and in the direction of the point vx, vy, vz.
Parameters:
vx, vy, vz: Numbers - The x, y, and z components of a vector from the origin (0,0,0) to the point vx,vy,vz. The vector can be of any length (it will be converted to unit length before use). The vector defines the axis, about which all points of the object will be rotated..
degs: Number - the angle by which this transform will rotate the path segments. The angle 'degs' is measured in degrees with rotation direction according to the 'right hand grip' rule (anti-clockwise if the axis vector is pointing out of the screen).
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
const newObj = obj.scale(scl);
Description:
The scale method multiplies all obj segments X,Y and Z coordinates by 'scl'. The scaling is centered on the drawing origin (0,0). The method returns a new Cgo3Dsegs array object containing the scaled coordinates. The original 'obj' remains unaltered.
Parameters:
scl: Number - A number greater than 0, by which the object's dimensions are scaled.
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
const newObj = obj.scaleNonUniform(xScale, yScale, zScale);
Description:
The scaleNonUniform method scales all obj X coordinates by a factor of 'xScale', the Y coordinates by a factor of 'yScale' and Z coordinates by a factor of 'zScale'. The method returns a new Cgo3Dsegs array object containing the original commands with the scaled coordinates. The original 'obj' is remains unaltered.
Parameters:
xScale, yScale, zScale: Numbers - Scale factors, greater than 0.
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Syntax:
const newObj = obj.translate(x, y, z);
Description:
The translate method adds the values x, y and z to all segment x,y,z coordinates respectively of a copy of obj. The copy is thus effectively shifted from its drawing origin (0,0,0) by x,y,z. The method returns a new Cgo3Dsegs object containing the translated segments. The original 'obj' remains unaltered.
Parameters:
x, y: Numbers - X, Y and Z offsets from the current drawing origin.
Returns:
Cgo3Dsegs object - suitable for use as the descriptor of an Shape3D or Panel3D object. The Cgo3Dsegs object returned has all the methods for further manipulation of the path data.
Text3D character set
ASCII characters
Character | Character | Character | Character | Character | Character | Character | Character |
---|---|---|---|---|---|---|---|
, | 8 | C | O | [ | g | s | |
! | - | 9 | D | P | \ | h | t |
" | . | : | E | Q | ] | i | u |
# | / | ; | F | R | ^ | j | v |
$ | 0 | < | G | S | _ | k | w |
% | 1 | = | H | T | ` | l | x |
& | 2 | > | I | U | a | m | y |
' | 3 | # | J | V | b | n | z |
( | 4 | ? | K | W | c | o | { |
) | 5 | @ | L | X | d | p | | |
* | 6 | A | M | Y | e | q | } |
+ | 7 | B | N | Z | f | r | ~ |
Special characters
To generate the symbols shown in columns 1, 3, 5 and 7, insert the Unicode in the adjacent column into the string.
Character | Unicode | Character | Unicode | Character | Unicode | Character | Unicode |
---|---|---|---|---|---|---|---|
Α | '\u0391' | Ξ | '\u039E' | α | '\u03B1' | ξ | '\u03BE' |
Β | '\u0392' | Ο | '\u039F' | β | '\u03B2' | ο | '\u03BF' |
Γ | '\u0393' | Π | '\u03A0' | γ | '\u03B3' | π | '\u03C0' |
Δ | '\u0394' | Ρ | '\u03A1' | δ | '\u03B4' | ρ | '\u03C1' |
Ε | '\u0395' | ° | '\u00B0' | ε | '\u03B5' | ÷ | '\u00F7' |
Ζ | '\u0396' | Σ | '\u03A3' | ζ | '\u03B6' | σ | '\u03C3' |
Η | '\u0397' | Τ | '\u03A4' | η | '\u03B7' | τ | '\u03C4' |
Θ | '\u0398' | ϒ | '\u03A5' | θ | '\u03B8' | υ | '\u03C5' |
Ι | '\u0399' | Φ | '\u03A6' | ι | '\u03B9' | φ | '\u03C6' |
Κ | '\u039A' | Χ | '\u03A7' | κ | '\u03BA' | χ | '\u03C7' |
Λ | '\u039B' | Ψ | '\u03A8' | λ | '\u03BB' | ψ | '\u03C8' |
Μ | '\u039C' | Ω | '\u03A9' | μ | '\u03BC' | ω | '\u03C9' |
Ν | '\u039D' | · | '\u00B7' | ν | '\u03BD' | × | '\u00D7' |
Colors:
Fill color, back color or stroke color parameters may be specified by a string representing a color in one of the CSS color formats.
CSS Color formats
There are five different formats that can be used to define a color for use in the canvas:
- 6 Digit RGB hex notation, '#rrggbb', where 'rr' sets the Red level (00 to ff), 'gg' sets the Green and 'bb' sets the Blue level.
- 3 Digit RGB hex notation '#rgb', this is converted into six-digit form (#rrggbb) by replicating digits, not by adding zeros. For example, #fb0 expands to #ffbb00.
- RGBA notation 'rgba(r, g, b, a)' where r, g and b are decimal numbers in the range 0 to 255 representing the Red, Green and Blue levels respectively and a is the Alpha or transparency value, 0 being fully transparent and 1.0 fully opaque.
- RGB notation "rgb(r, g, b)" where r, g and b are decimal numbers in the range 0 to 255 representing the red, green and blue levels respectively. This is a suitable format for entering dynamic color values. The calculated r, g and b values may be passed to drawing methods by the string 'rgb('+r+','+g+','+b+')'.
- Predefined colors names specified by the Extended CSS Color List eg. 'red', 'blue', 'maroon', 'palegoldenrod', 'wheat' etc. There are 143 standard colors named in the list and 'transparent' is also supported.